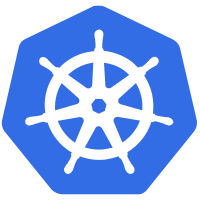
My Laravel site was working just fine yesterday but after a code-only update today I was seeing 502 errors on some pages
upstream sent too big header while reading response header from upstream, client:
While the solution was hard to find it was easy to implement.
Read more ...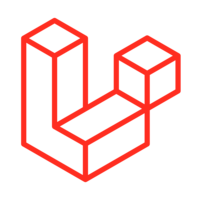
Yesterday I thought I’d fixed my https links on Laravel running in Kubernetes - but I had a nagging feeling that I’d just followed some random blog posts and missed something …
Unfortunately what I had looked for was force laravel to use https
When what I really needed was Configuring Trusted Proxies
Read more ...
Read Laravel HTTPS Behind a Proxy instead
The post below is wrong
This doesn’t seem to be well documented - I cant find anything about it in the official docs.
Thanks to Md Obydullah at shouts.dev
My Laravel site runs in kubernetes where TLS encryption happens in a proxy layer and I need Laravel to server content with https links.
Read more ...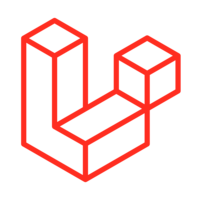
As a backend developer I’m a big fan of Bootstrap it is a framework that lets me quickly put together user interfaces with consistent components using Modals, Alerts, Toasts and more - things that I don’t want to have to learn how to make work and add design to.
It seems like Laravel used to use Bootstrap by default but has migrated to Tailwind CSS
Read more ...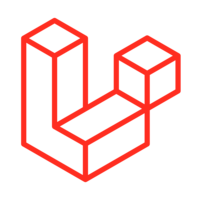
Laravel has some really good features for setting database connections - but oddly this isn’t spelled out in the documentation.
Databases (especially in Docker containers) often come by default with a single, powerful, user account.
As a result all too often people run Laravel without considering the principle of least privilege.
By following a few simple steps we can enhance security.
Read more ...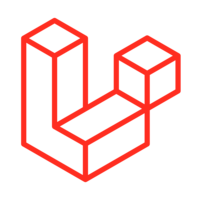
Laravel’s database migrations is a great system and makes it easy for the development team to stay in sync with schema changes as well as ensuring tests can run against a defined database state.
It also makes great use of transactions to efficiently roll back changes after each test
But what if you have some large tables of fairly static data that you don’t want to reload on every test run …
Read more ...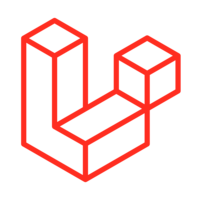
Something didn’t quite click with me about Laravel Eloquent Models.
There is nothing in the Model that defines the fields.
The Model defines which database table the data is stored in.
Whatever fields are in the table will be loaded to the Model.
Read more ...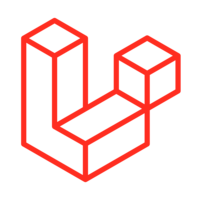
I wanted to better understand what is happening when I run Laravel tests that hit the database.
TLDR: Database migrations are run on every test run, optionally with seed data.
Each test case runs in a transaction.
(This is written based on Laravel 9)
Read more ...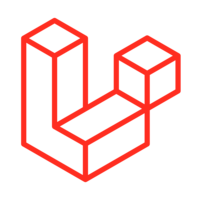
How to find out what version of Laravel you have.
The difference between a laravel-framework update and a laravel skeleton update.
What is Laravel skeleton anyway ?
How to control when you get updates.
Read more ...Laravel’s routing and binding is nice
But when I got it wrong I didn’t get the kind of error I expected
Laravel automatically resolves Eloquent models defined in routes or controller actions whose type-hinted variable names match a route segment name.
use App\Models\User;
Route::get('/users/{user}', function (User $user) {
return $user->email;
});
Read more ...